Developer Language
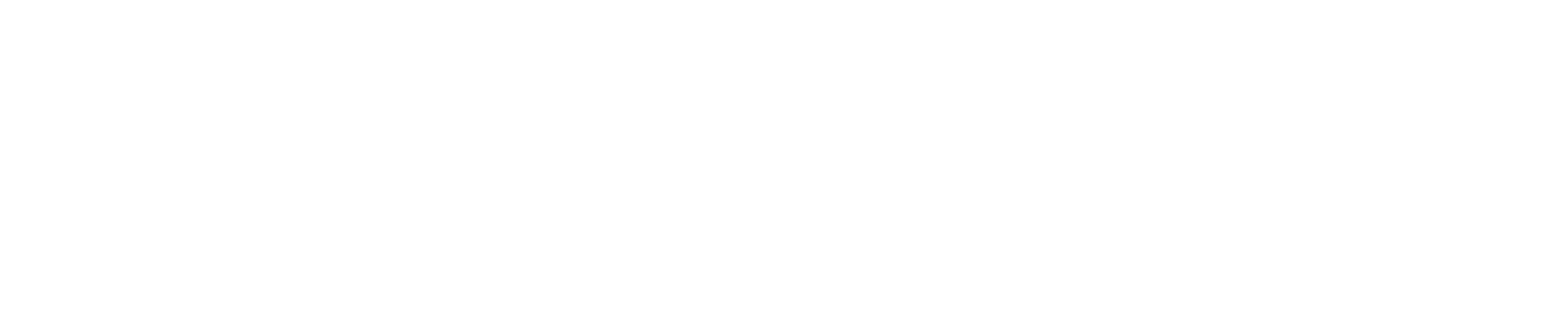
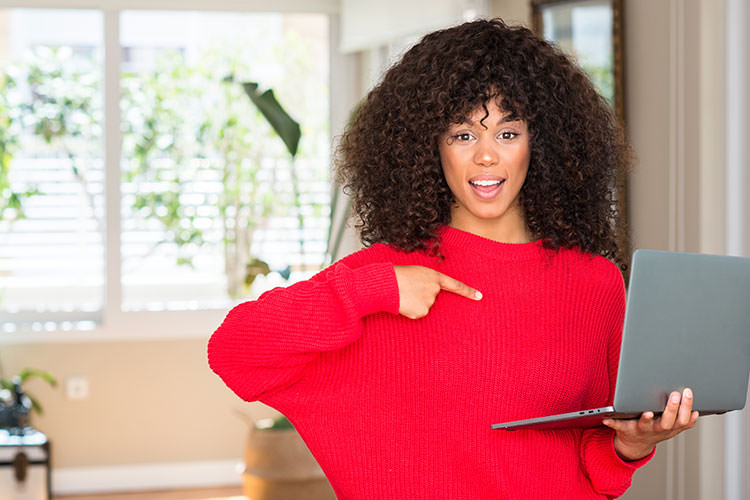
Developer looking for language?
C++ SMS API
Use this API to send SMS with C++.
- Easy-to-use DLL
- Delivery status via function return code
- Email replies
- Proxy server support
Example:
int SendSMS(char *AccountID, char *EmailAddress, char *Password, char *Recipient, char *Message)
{
HMODULE RedOxygenLibrary;
SendSMSFunction *SendSMS;
int Result;
RedOxygenLibrary = LoadLibrary(“redoxygen.dll”);
SendSMS = (SendSMSFunction *) GetProcAddress(RedOxygenLibrary, “SendSMS”);
Result = SendSMS(AccountID, EmailAddress, Password, Recipient, Message);
FreeLibrary(RedOxygenLibrary);
return Result;
}
Send SMS with C#
Use this SMS gateway API to send SMS with C# from your PC. This simple but powerful C# SMS API builds SMS sending and receiving functionality into your existing communication infrastructure. Begin with the Core API. If you discover there are other capabilities to send SMS with C# you’re desiring – such as bulk messaging, scheduled deliveries, etc. – simply use the Extended API. Contact us for more technical details.
Features
- Simple HTTP request
- Delivery status via function return code
- Replies to your SMS arrive back as emails
Example:
public int SendSMS(String AccountID, String Email, String Password, String Recipient, String Message)
{
WebClient Client = new WebClient();
String RequestURL, RequestData;
RequestURL = “https://redoxygen.net/sms.dll?Action=SendSMS”;
RequestData = “AccountId=” + AccountID
+ “&Email=” + System.Web.HttpUtility.UrlEncode(Email)
+ “&Password=” + System.Web.HttpUtility.UrlEncode(Password)
+ “&Recipient=” + System.Web.HttpUtility.UrlEncode(Recipient)
+ “&Message=” + System.Web.HttpUtility.UrlEncode(Message);
byte[] PostData = Encoding.ASCII.GetBytes(RequestData);
byte[] Response = Client.UploadData(RequestURL, PostData);
String Result = Encoding.ASCII.GetString(Response);
int ResultCode = System.Convert.ToInt32(Result.Substring(0, 4));
return ResultCode;
}
Delphi SMS API
Use this API to send SMS from Delphi.
- Easy-to-use DLL
- Delivery status via function return code
- Email replies
- Proxy server support
Example:
function SendSMS (
AccountId : String;
Email : String;
Password : String;
Recipient : String;
Message : String) : Integer; stdcall; external ‘redoxygen.dll’;
procedure SendMessage (AccountID, Email, Password, Recipient, Message : String)
var
Result : Integer;
begin
Result := SendSMS(AccountID, Email, Password, Recipient, Message);
end;
Java SMS API
Use this SMS gateway API to send SMS using Java from your PC. This simple but powerful Java SMS API works with your messaging existing application to enable the capabilities to receive and send SMS. Everyone begins with the Core API. If you’re looking for additional functionality (e.g. scheduled message deliveries, bulk messaging, etc.), download the Extended API. Visit here for additional technical details. To get started with this Java SMS API, contact us today.
Features
- Simple HTTP request
- Communications encrypted with SSL
- Message delivery status via function return code
- Replies to your outbound SMS arrive to you as email
Example:
public static int SendSMS(String AccountID, String Email, String Password,
String Recipient, String Message, StringBuffer Response)
{
String RequestURL = “http://www2.redoxygen.net/sms.dll?Action=SendSMS”;
String Data = (“AccountId=” + URLEncoder.encode(AccountID, “UTF-8”));
Data += (“&Email=” + URLEncoder.encode(Email, “UTF-8”));
Data += (“&Password=” + URLEncoder.encode(Password, “UTF-8”));
Data += (“&Recipient=” + URLEncoder.encode(Recipient, “UTF-8”));
Data += (“&Message=” + URLEncoder.encode(Message, “UTF-8”));
int Result = -1;
URL Address = new URL(RequestURL);
HttpURLConnection Connection = (HttpURLConnection) Address.openConnection();
Connection.setRequestMethod(“POST”);
Connection.setDoInput(true);
Connection.setDoOutput(true);
DataOutputStream Output;
Output = new DataOutputStream(Connection.getOutputStream());
Output.writeBytes(Data);
Output.flush();
Output.close();
BufferedReader Input = new BufferedReader(new InputStreamReader(Connection.getInputStream()));
StringBuffer ResponseBuffer = new StringBuffer();
String InputLine;
while ((InputLine = Input.readLine()) != null)
{
ResponseBuffer = ResponseBuffer.append(InputLine);
ResponseBuffer = ResponseBuffer.append(“\n\n\n”);
}
Response.replace(0, 0, ResponseBuffer.toString());
String ResultCode = Response.substring(0, 4);
Result = Integer.parseInt(ResultCode);
Input.close();
return Result;
}
Perl SMS API
Use this API to send SMS from Perl.
- Simple HTTP request
- SSL-encrypted communication
- Delivery status via function return code
- Email replies
Example:
sub SendSMS
{
my $Browser = LWP::UserAgent->new;
(my $AccountID, my $Email, my $Password, my $Recipient, my $Message) = @_;
my $URL = ‘http://www2.redoxygen.net/sms.dll?Action=SendSMS’;
my $Response = $Browser->post($URL,
[‘AccountID’ => $AccountID,
‘Email’ => $Email,
‘Password’ => $Password,
‘Recipient’ => $Recipient,
‘Message’ => $Message]);
print $Response->content;
}
Send SMS with PHP
Use this SMS gateway API to send SMS with PHP from your computer. This PHP SMS API is simple but powerful integration, enabling the capabilities to receive and send SMS with PHP from your existing messaging infrastructure. You’ll begin with the Core API. If you find you’d enjoy additional features – like bulk SMS distributions or scheduled message deliveries, for instance – simply use the Extended API. For more technical details, contact us today.
Features
- Simple HTTP request
- Communications secured with SSL encryption
- Delivery status of message via function return code
- Replies to your outbound SMS arrive back as emails
Example:
function SendMessage($AccountID, $Email, $Password, $Recipient, $Message)
{
$Parameters[‘AccountID’] = $AccountID;
$Parameters[‘Email’] = $Email;
$Parameters[‘Password’] = $Password;
$Parameters[‘Recipient’] = $Recipient;
$Parameters[‘Message’] = $Message;
Request($Parameters, ‘http://sms1.redoxygen.net/sms.dll?Action=SendSMS’);
}
function Request($Parameters, $URL)
{
$URL = preg_replace(‘@^http://@i’, ”, $URL);
$Host = substr($URL, 0, strpos($URL, ‘/’));
$URI = strstr($URL, ‘/’);
$Body = ”;
foreach($Parameters as $Key => $Value)
{
if (!empty($Body))
{
$Body .= ‘&’;
}
$Body .= $Key . ‘=’ . urlencode($Value);
}
$ContentLength = strlen($Body);
$Header = “POST $URI HTTP/1.1\n”;
$Header .= “Host: $Host\n”;
$Header .= “Content-Type: application/x-www-form-urlencoded\n”;
$Header .= “Content-Length: $ContentLength\n\n”;
$Header .= “$Body\n”;
$Socket = fsockopen($Host, 80, $ErrorNumber, $ErrorMessage);
fputs($Socket, $Header);
while (!feof($Socket))
{
$Result[] = fgets($Socket, 4096);
}
fclose($Socket);
}
Visual Basic SMS API
Use this API to send SMS from Visual Basic.
- Simple HTTP request
- Encrypted communication over SSL
- Long SMS (up to 765 characters)
- Delivery status via function return code
- Email replies
Example:
Public Shared Function SendSms(ByVal AccountID As String, ByVal Email As String, ByVal Password As String,
ByVal Recipient As String, ByVal Message As String) As Integer
Dim Client As WebClient = New WebClient
Dim URL As String
URL = (“http://www.redoxygen.net/sms.dll?Action=SendSMS” _
+ “&AccountId=” + AccountID _
+ “&Email=” + Email _
+ “&Password=” + Password _
+ “&Recipient=” + Recipient _
+ “&Message=” + Message)
Dim Response() As Byte = Client.DownloadData(URL)
Dim Result As String = Encoding.ASCII.GetString(Response)
Dim ResultCode As Integer = System.Convert.ToInt32(Result.Substring(0, 4))
Return ResultCode
End Function