Developer Interface
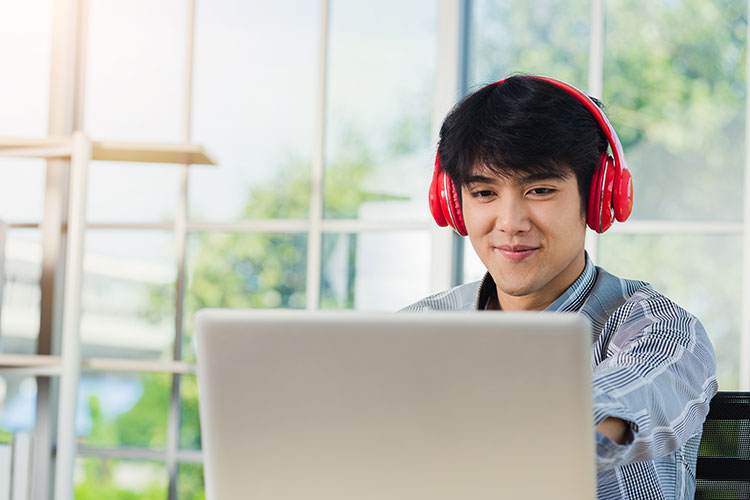
What Interface Are You Using?
Command Line SMS API
Use this API to send SMS from a command line.
- Easy configuration via an INI file
- Send SMS from scripts and batch files
- Firewall friendly
- XCOPY deployment
- Compatible with Microsoft Windows 7, 8, 8.1, & 10 and Windows Server 2008+
Example:
[CONFIG]
AccountId=
Email=
Password=
[PROXY]
Server=
Port=
Username=
Password=
C:\> SendSMS “0410123456, 0410654321” “SMS message to multiple recipients”
COM SMS API
Use this API to send SMS via a COM component.
- User-friendly API
- Proxy server support
- Delivery status via function return code
- Replies to your SMS arrive to you as email
Example:
Dim RedOxygenComponent As Object
Set RedOxygenComponent = CreateObject(“REDOXYGENCOM.SMSInterface”)
With RedOxygenComponent
.AccountID = “CI00001234”
.Email = “username@company.com”
.Password = “MyPassword”
End With
RedOxygenComponent.SendSMSMessage “0410123456”, “Hello from Red Oxygen COM object”
DLL SMS API
Use this API to send SMS via a linked library.
- Easy to use API
- DLL functions can be called from many languages
- Proxy server support
- XCOPY deployment
- Email replies
Example:
int SendSMS(char *AccountID, char *EmailAddress, char *Password, char *Recipient, char *Message)
{
HMODULE RedOxygenLibrary;
SendSMSFunction *SendSMS;
int Result;
RedOxygenLibrary = LoadLibrary(“redoxygen.dll”);
SendSMS = (SendSMSFunction *) GetProcAddress(RedOxygenLibrary, “SendSMS”);
Result = SendSMS(AccountID, EmailAddress, Password, Recipient, Message);
FreeLibrary(RedOxygenLibrary);
return Result;
}
Email SMS API
Use this API to send SMS via email.
- Automatic conversion from email into SMS
- IP or password authentication
- Send multiple SMS per email
- Replies to email or cell phone
Example:
To: 0410123456@redoxygen.netFrom: sms-alerts@company.com
Subject: Alert!
Body:
[SENDER]
ID=CI00012345
PW=password
Server 23 is offline.
SMS HTTP API
Use this SMS gateway API to send SMS via HTTP connection from your computer. This SMS HTTP API is simple but powerful; building in the functionality to receive and send SMS into your existing communication application. You’ll start with the Core API. If you find you’d like even more features (e.g. scheduled message deliveries, bulk messaging, etc.), simply download the Extended API. For additional technical details, visit here. Get started with this SMS HTTP API today, contact us.
Features
- Encrypted communication with SSL
- Replies from SMS recipients arrive to you as email
- Delivery status via function return code
- Multiple recipients per request
Example:
public int SendSMS(String AccountID, String Email, String Password, String Recipient, String Message)
{
WebClient Client = new WebClient();
String RequestURL, RequestData;
RequestURL = “https://redoxygen.net/sms.dll?Action=SendSMS”;
RequestData = “AccountId=” + AccountID
+ “&Email=” + System.Web.HttpUtility.UrlEncode(Email)
+ “&Password=” + System.Web.HttpUtility.UrlEncode(Password)
+ “&Recipient=” + System.Web.HttpUtility.UrlEncode(Recipient)
+ “&Message=” + System.Web.HttpUtility.UrlEncode(Message);
byte[] PostData = Encoding.ASCII.GetBytes(RequestData);
byte[] Response = Client.UploadData(RequestURL, PostData);
String Result = Encoding.ASCII.GetString(Response);
int ResultCode = System.Convert.ToInt32(Result.Substring(0, 4));
return ResultCode;
}